In this tutorial you will learn about difference between system software and application software.
System software is general purpose software which is used to operate computer hardware. It provides platform to run application softwares.
Application software is specific purpose software which is used by user for performing specific task.
Below I have shared some main differences between them.
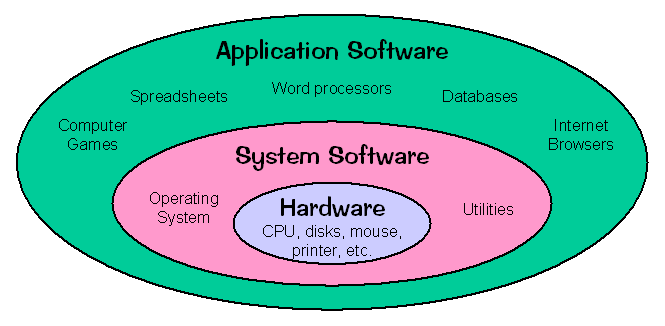
Comment below if you found anything incorrect in above difference between system software and application software tutorial. Please mention below if you know about any other difference.
System software is general purpose software which is used to operate computer hardware. It provides platform to run application softwares.
Application software is specific purpose software which is used by user for performing specific task.
Below I have shared some main differences between them.
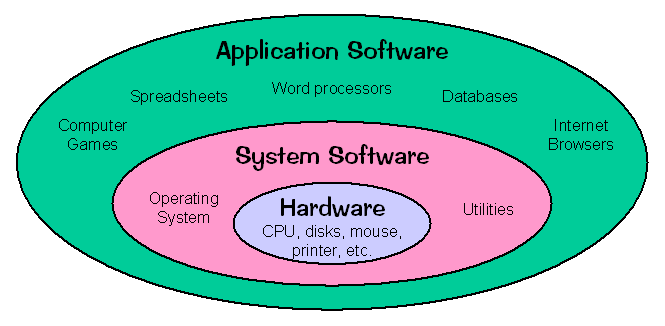
Difference between System Software and Application Software
S.No. | System Software | Application Software |
1. | System software is used for operating computer hardware. | Application software is used by user to perform specific task. |
2. | System softwares are installed on the computer when operating system is installed. | Application softwares are installed according to user’s requirements. |
3. | In general, the user does not interact with system software because it works in the background. | In general, the user interacts with application sofwares. |
4. | System software can run independently. It provides platform for running application softwares. | Application software can’t run independently. They can’t run without the presence of system software. |
5. | Some examples of system softwares are compiler, assembler, debugger, driver, etc. | Some examples of application softwares are word processor, web browser, media player, etc. |
Comment below if you found anything incorrect in above difference between system software and application software tutorial. Please mention below if you know about any other difference.